We'll start by putting the flag down.
In the 2D view, right click at the point
shown and select team/team_wolf_checkpoint.
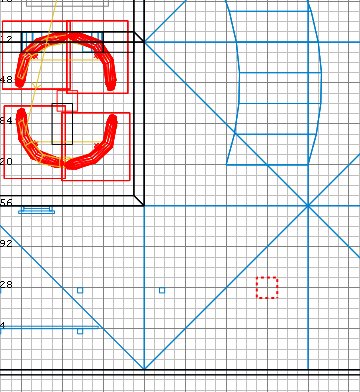
In the side 2D view, move the entity
until its bottom just about goes into the
ground. Be aware that if you put a
flag on a rooftop, the rolled up flag of the
losing team will appear below the bottom of
the flagpole, which might appear to be
hanging from the ceiling of the room
below...
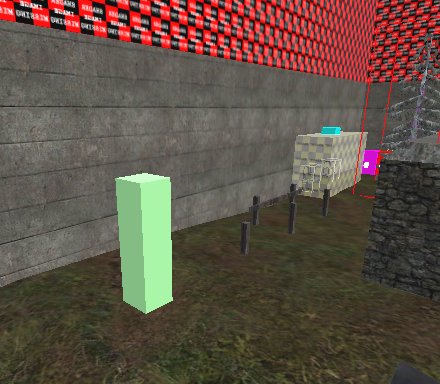
Press N. Close the window and press
N again.
Tick the spawnpoint box.
We need to provide a name for the entity
so we can have a script procedure for
it. Enter a targetname of forward_flag
and then a scriptname also of forward_flag.
The name can be anything of course, but
we'll use forward_flag for now. This
value is not shown to players.
Finally we need to tell the flag the
names of the spawn locations that it is
controlling: enter a target of forward_spawns.
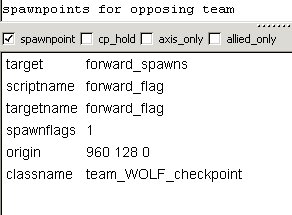
Ok, now we'll add the spawn points for
both teams. We'll add just one of each
to illustrate the technique - but you would
need to add 32 for each side.
Right-click in the 2D overhead view where
you want the first allied soldier to arrive,
and select team/team_ctf_bluespawn.
In the side view make sure the entity is on
or just above the ground. For
irregular surfaces like terrain, make it
just above, otherwise he'll start with his
feet in the ground and not be able to move.
Press N, and tick the invulnerable
box. I think this may be redundant but
what the heck. We won't tick the startactive
box because we don't want him spawning here
yet.
Click on one of the 8 directional buttons
at bottom left of the Entities window, to
set the direction the player will be looking
in on spawning.
Enter a targetname of forward_spawns
and close the entities window. If you
have done everything right, the flag will
now have a line drawn to the blue spawn
entity. Press ESC.
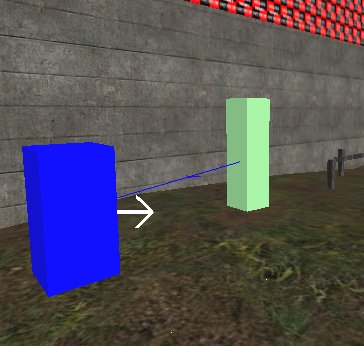
Now right-click in the 2D overhead view
where you want the Axis soldier to
spawn. If space is tight, the axis
spawns can overlap the allied spawns.
Select team/team_ctf_redpawn and
position it clear of the ground and press N.
Now do the same things that you did for
the blue spawn, that is, set the invulnerable
box and give it the same targetname,
and any facing direction you want.
Close the window and press ESC.
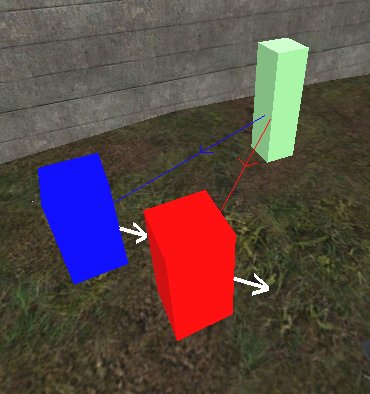
You should have a red line connecting the
flag to the spawn.
Finally we need to add the command map
marker entity to show where the flag
is. Usually you put this over the flag
entity. So right-click on the flag
entity in the overhead view and select team/team_wolf_objective.
Position the entity a little over the flag.
Press N. There has to be a
default owner, even if really there is
none. So tick the default_axis
box. We'll take care of the real
situation in the script.
Enter a description of Forward
Flag - this text will be shown on the
command map. Enter a targetname
and scriptname of forward_wobj.
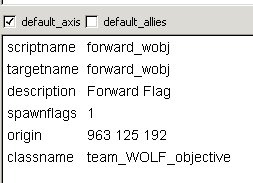
Close the window and press ESC. We
have completed the mapping element.
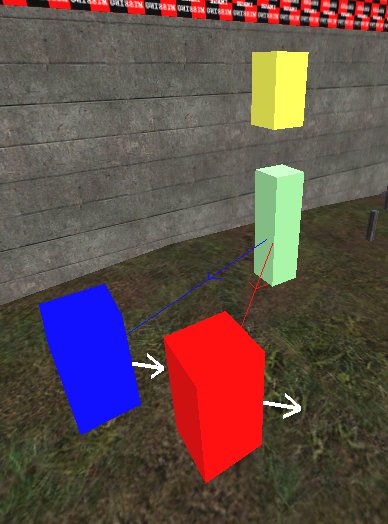
Save the map and compile it. Don't
run ET yet.
|